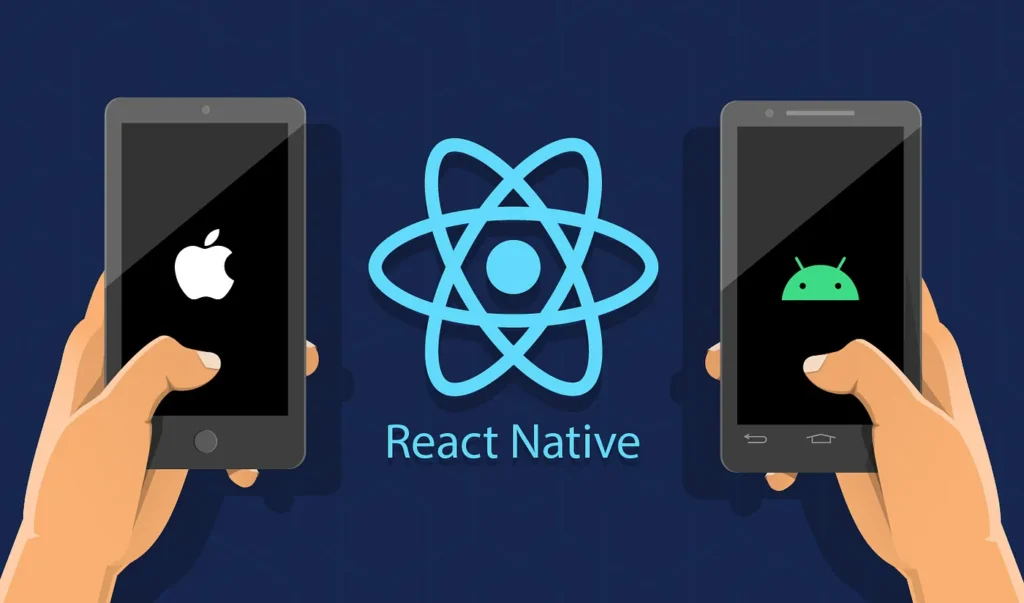
Introduction
React Native is a popular open-source framework developed by Meta for building cross-platform mobile applications. Leveraging the power of React, it allows developers to create native-like experiences for both iOS and Android platforms using a single codebase, written primarily in JavaScript and JSX.
React Native is widely used by developers and organizations for various purposes:
- Cross-Platform Development: React Native enables developers to write code once and deploy it across multiple platforms, saving time and resources.
- Community Support: React Native has a wide range of community of developers contributing to its ecosystem, providing libraries, tools, and resources to simplify development tasks.
- Native-Like Performance: By rendering UI components using native APIs, React Native apps provide a smooth and responsive user experience similar to that of native applications.
- Cost-Effectiveness: Developing apps with React Native can be more cost-effective compared to building separate native apps for iOS and Android platforms.
Opimization Techniques
React Native provides developers with a powerful framework for building cross-platform mobile applications, but ensuring optimal performance is crucial for delivering a smooth and responsive user experience. In this section, we’ll explore various performance considerations and optimization techniques for React Native apps.
- Minimizing Re-renders with Memoization: React Native re-renders components when their state or props change. To optimize performance, use memoization techniques like
React.memo
for functional components orshouldComponentUpdate
for class components. This prevents unnecessary re-renders, improving app performance. - Virtualized Lists for Efficient Rendering: When dealing with large datasets or lists, utilize virtualized lists such as
FlatList
andSectionList
. These components efficiently render only the items that are currently visible on the screen, reducing memory usage and improving rendering performance. - Optimize Image Loading: Loading and rendering images can significantly impact app performance, especially when dealing with large images. Use libraries like
react-native-fast-image
to optimize image loading by leveraging features such as image caching, lazy loading, and progressive loading
npm i react-native-fast-image
or
yarn add react-native-fast-image
import FastImage from 'react-native-fast-image';
- Bundle Size Optimization: Reducing the size of the app bundle is essential for faster app startup times and improved performance. Employ techniques like code splitting, tree shaking, and dynamic imports to eliminate unused code and dependencies, resulting in a smaller bundle size.
- Memory Management: Proper memory management is crucial for preventing memory leaks and optimizing app performance. Utilize tools like React Native Debugger and Chrome DevTools for memory profiling, identify memory-intensive components, and optimize their usage to minimize memory footprint.
- Network Optimization: Efficient network requests are essential for responsive app performance. Implement techniques like caching network responses, optimizing API requests, and using libraries like
axios
with built-in request cancellation and batching capabilities to optimize network performance.
npm i axios
import axios from "axios";
const handleUploadAll = async () => {
const apiUrl =
'back-end-api';
const batchSize = 10;
const totalItems = tableData.length;
let uploadSuccess = true;
setLoading(true);
try {
for (let i = 0; i < totalItems; i += batchSize) {
const batch = tableData.slice(i, i + batchSize);
const payload = {
scans: batch,
};
const response = await axios.post(apiUrl, payload, {
headers: {
'Content-Type': 'application/json',
},
});
if (response.status !== 200) {
uploadSuccess = false;
break;
}
}
if (uploadSuccess) {
clearData();
Alert.alert('Data Uploaded');
}
} catch (error) {
Alert.alert('Something went wrong');
} finally {
setLoading(false);
}
};
- Native Module Optimization: If your app utilizes native modules, ensure they are optimized for performance. Minimize unnecessary bridge calls between JavaScript and native code, optimize native module implementation, and utilize asynchronous APIs to prevent blocking the JavaScript thread.
Optimizing React Native app performance requires a combination of best practices, optimization techniques, and performance testing. By implementing these strategies, developers can create high-performing React Native apps that deliver a seamless and responsive user experience across platforms.
Remember to continually monitor and optimize app performance as your app evolves to ensure optimal performance under different usage scenarios and device conditions.
React Native Best Practices
- Use Functional Components and Hooks: Functional components offer a more concise syntax and better performance compared to class components. Hooks like
useState
anduseEffect
simplify state management and side effects, leading to cleaner and more maintainable code. - Follow Component Modularization: Divide your app into smaller, reusable components that encapsulate specific functionalities. This promotes code reusability, readability, and easier maintenance of the application.
- Implement Virtualized Lists with FlatList: When rendering large lists or grids, use the
FlatList
component to efficiently render only the items that are currently visible on the screen. This reduces memory usage and improves rendering performance by avoiding the rendering of off-screen items. - Minimize Redux Usage with Context API: While Redux is a powerful state management tool, it can introduce unnecessary complexity for smaller applications. Consider using the Context API for managing global state when Redux is not required, as it offers a simpler and more lightweight alternative. Redux is more reliable with react web applications.
- Use React.memo for Performance Optimization: Wrap your components with
React.memo
to prevent unnecessary re-renders. These optimizations compare the current props with the previous props and shallowly compare state changes to determine if a re-render is necessary, thereby improving performance. - Optimize Navigation with React Navigation: When implementing navigation in your React Native app, use a library like React Navigation that offers a flexible and performant navigation solution. React Navigation provides various navigators optimized for different use cases, ensuring smooth and efficient navigation transitions.
- Avoid Inline Styles, Use StyleSheet: Instead of applying styles inline, use the
StyleSheet
API provided by React Native to define styles separately. StyleSheet optimizes styles by caching them and applying them more efficiently, resulting in improved performance compared to inline styles. But some special special cases you have to use inline stylling as well.
Conclusion
In conclusion, optimizing React Native app performance is important for delivering a seamless and responsive user experience across mobile devices. By adhering to best practices such as using functional components and hooks, modularizing components, and implementing performance optimizations like virtualized lists and image loading enhancements, developers can significantly improve the performance of their apps. Additionally, utilizing libraries like React Navigation and axios for navigation and network requests respectively, along with proper memory management and native module optimization, further enhances app performance. By following these best practices and continuously monitoring app performance, developers can ensure their React Native apps are not only efficient and performant but also deliver a delightful user experience to their audience.